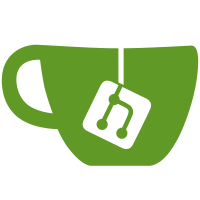
5 changed files with 539 additions and 284 deletions
@ -0,0 +1,216 @@
@@ -0,0 +1,216 @@
|
||||
function TurkeyLayer( name, percentRadius, turkeyModel, ovenModel ){ |
||||
var that = this; |
||||
|
||||
this.name = name; |
||||
this.initialTemp = 20; |
||||
this.waterContent = 100000; |
||||
this.Qdot = 0; |
||||
this.finalTemperature = 20; |
||||
|
||||
return { |
||||
updateTemperatureTick: function(){ |
||||
that.finalTemperature = UtilityFunctions.transientSphereSeries( turkeyModel.density, |
||||
turkeyModel.thermalConduct, |
||||
turkeyModel.heatConvection, |
||||
turkeyModel.cp, |
||||
percentRadius * turkeyModel.totalRadius, |
||||
turkeyModel.totalRadius, |
||||
that.initialTemp, |
||||
ovenModel.tempInfini, |
||||
globalTime ); |
||||
that.initialTemp = that.finalTemperature; |
||||
} |
||||
} |
||||
} |
||||
|
||||
function TurkeyModel( weight, ovenModel ){ |
||||
this.density = 996; // kg/m3 Assuming Density of Water 1000 kg/m3
|
||||
this.cp = 2810; // J/kg K for Turkey
|
||||
this.heatConvection = 5; // W/m2 K Some Reasonable estimate for natural Convection. Change as needed. 5-25
|
||||
this.thermalConduct = 0.412; // W/m K // Chicken
|
||||
|
||||
this.totalRadius = UtilityFunctions.calculateRadius( weight, this.density ); |
||||
this.totalLayers = [ new TurkeyLayer("Skin", 0.85, this, ovenModel ), |
||||
new TurkeyLayer("Body", 0.45, this, ovenModel ), |
||||
new TurkeyLayer("Core", 0.01, this, ovenModel ) ]; |
||||
|
||||
// Whenever temperature is changed
|
||||
this.updateLayerTemps = function() { |
||||
for (var i in this.totalLayers ){ |
||||
this.totalLayers[i].updateTemperatureTick(); |
||||
} |
||||
} |
||||
} |
||||
|
||||
function OvenModel() { |
||||
this.tempInfini=20; //C
|
||||
this.setTemp = 20; |
||||
|
||||
var proportional = 0.1; // This value is arbitrary to how fast you want the temperatures to converge. (Or oscillate, which could be realistic as well)
|
||||
var errorTolerance = 5; //Stove is accurate to 1 degree Celcius Should hopefully oscillate below that value.
|
||||
|
||||
this.changeTemp = function(setTemp) { |
||||
this.setTemp = setTemp; |
||||
} |
||||
|
||||
// Equalize temp will need to be sent each time iteration
|
||||
this.equalizeTemp = function() { |
||||
var error = Math.abs(this.setTemp-this.tempInfini); |
||||
if( this.setTemp>this.tempInfini ){ |
||||
this.tempInfini = this.tempInfini + error*proportional; |
||||
} |
||||
else if( this.setTemp<this.tempInfini ){ |
||||
this.tempInfini = this.tempInfini - error*proportional; |
||||
} |
||||
|
||||
if( error>errorTolerance ) { |
||||
return (true) //Need to run the Heat Calculations again next cycle
|
||||
} |
||||
} |
||||
} |
||||
|
||||
|
||||
UtilityFunctions = { |
||||
|
||||
// Cache the lambda if the Biot number does not change, to avoid expensive root-finding operations
|
||||
cachedBiot: null, |
||||
cachedLambda: null, |
||||
|
||||
// Using Ratios for a rectangular Box Turkey
|
||||
calculateRadius: function(weight, density) { |
||||
|
||||
var ratioLvG=1.4; //1.4, Turkey length vs shoulder girth
|
||||
var ratioLvH=2; //2, Turkey length vs height from resting position
|
||||
|
||||
var length = Math.pow(weight/((1/ratioLvG)*(1/ratioLvH)*density),(1/3)) |
||||
var depth = 1/(ratioLvG /length); |
||||
var height = 1/(ratioLvH /length); |
||||
var simpleRadius = length/2; //Doesn't take into account equal Volume
|
||||
|
||||
var rectangleVolume = depth*height*length*(1/3); //m^3 Multiple by 1/3 to account for triangular shape and empty Space
|
||||
var complexRadius = Math.pow(rectangleVolume/((4/3)*Math.PI), 1/3); //Volume of 3D Box = 3D Sphere
|
||||
|
||||
console.log("Simple Radius " + simpleRadius + " Meters") |
||||
console.log("Complex Radius " + complexRadius + " Meters") |
||||
return complexRadius; |
||||
}, |
||||
|
||||
findAllRoots: function(min,max,splitsNum,Biot) { |
||||
var step = ( max - min ) / ( splitsNum - 1 ); |
||||
var answer; |
||||
var negativeTest; |
||||
var storage = []; |
||||
for (var i = step; i < max; i=i+step ) { |
||||
negativeTest = this.lambdaFormula(i-step, Biot)*this.lambdaFormula(i, Biot); |
||||
if (negativeTest <= 0) { |
||||
answer = this.bisectionMethod(i-step,i,Biot); |
||||
if (answer !=undefined) { |
||||
storage.push(answer); |
||||
} |
||||
} |
||||
else { |
||||
//console.log("No Bracketed Root " + negativeTest)
|
||||
} |
||||
} |
||||
return storage; |
||||
}, |
||||
|
||||
bisectionMethod: function(min,max,Biot) { |
||||
errorTolerance = (1/Math.pow(10,8)) |
||||
result = Infinity // some large value to ensure the calculation goes through.
|
||||
negativeTest =this.lambdaFormula(min, Biot)*this.lambdaFormula(max, Biot) |
||||
if (negativeTest <=0 ) { |
||||
var antiFreeze=0; |
||||
while (Math.abs(result) > errorTolerance && antiFreeze<=500) { //The greater the antiFreeze, the more wasted cycles around a singularity
|
||||
lambdaN = (min+max)/2 |
||||
result=this.lambdaFormula(lambdaN, Biot) |
||||
if (Math.abs(result) <= errorTolerance && result<=errorTolerance) { |
||||
return (lambdaN); //At Root
|
||||
} |
||||
else if ((this.lambdaFormula(min, Biot)*this.lambdaFormula(lambdaN, Biot))>=0) { |
||||
min=lambdaN; |
||||
} |
||||
else if ((this.lambdaFormula(max, Biot)*this.lambdaFormula(lambdaN, Biot))>=0) { |
||||
max=lambdaN; |
||||
} |
||||
antiFreeze++ |
||||
} |
||||
} |
||||
}, |
||||
|
||||
lambdaFormula: function( lambdaN, Biot ) { |
||||
var result = 1-lambdaN*(1/Math.tan(lambdaN))-Biot; |
||||
return(result) |
||||
}, |
||||
|
||||
transientSphereSeries: function( density, thermalConduct, heatConvection, cp, rPosition, rTotal, tempInitial, tempInfini, t ){ |
||||
var min = 0; |
||||
var max = 1000; // This are for setting Lambda boundaries and nothing else
|
||||
|
||||
var sum=0; |
||||
var alpha = thermalConduct/(density*cp); |
||||
var lambdaN; |
||||
var sinPortion; |
||||
var exponentialPortion; |
||||
var frontCoefficientPortion; |
||||
|
||||
|
||||
//console.log("Alpha is " + alpha)
|
||||
|
||||
var Fourier = (alpha*t)/Math.pow(rTotal,2) |
||||
//console.log("Fourier is " + Fourier)
|
||||
|
||||
var biotNum = heatConvection * rTotal/thermalConduct |
||||
|
||||
if ( biotNum != this.cachedBiot ) { |
||||
console.log("Recalculating Lambda Terms") |
||||
this.cachedLambda = this.findAllRoots(min,max,max*Math.PI*10,biotNum) |
||||
this.cachedBiot = biotNum; |
||||
} |
||||
|
||||
//console.log("The Biot Value is " + biotNum)
|
||||
|
||||
for (var i = 0; i<this.cachedLambda.length; i++) { |
||||
var lambdaN = this.cachedLambda[i]; |
||||
var sinPortion= Math.sin(lambdaN*rPosition/rTotal)/(lambdaN*rPosition/rTotal); |
||||
var exponentialPortion = (1/Math.exp(Math.pow(lambdaN,2)*Fourier)); |
||||
var frontCoefficientPortion = 4*(Math.sin(lambdaN)-(lambdaN*Math.cos(lambdaN)))/ (2*lambdaN-Math.sin(2*lambdaN)); |
||||
sum = frontCoefficientPortion*exponentialPortion*sinPortion + sum; |
||||
} |
||||
|
||||
tempAtTimeAndRadius=(sum*(tempInitial-tempInfini))+tempInfini |
||||
|
||||
console.log("The Temperature at radius " + rPosition + " m and time " + t + " seconds is " + tempAtTimeAndRadius + " C or " + this.C2F(tempAtTimeAndRadius) + " F"); |
||||
return(tempAtTimeAndRadius) |
||||
}, |
||||
|
||||
/* Utility Functions */ |
||||
C2F: function( celsius ){ |
||||
return ( (celsius*(9/5)) + 32 ); |
||||
}, |
||||
F2C: function( farenheit ) { |
||||
return ( (farenheit*(9/5)) + 32 ); |
||||
}, |
||||
lbs2kgs: function(){ |
||||
return pounds * 0.453592 |
||||
} |
||||
} |
||||
|
||||
//Running the Program Stuff
|
||||
|
||||
var ovenObject = new OvenModel(); |
||||
var turkey = new TurkeyModel( 8, ovenObject ); |
||||
|
||||
globalTime=0; |
||||
setInterval(function(){time()},100); |
||||
|
||||
function time() { |
||||
console.clear() |
||||
if (ovenObject.equalizeTemp() ) { |
||||
globalTime = 0; //Reset the model's time calculation if there are major changes in the tolerance of the temperature
|
||||
} |
||||
else {globalTime = globalTime +60 } |
||||
console.log( ovenObject.tempInfini ) |
||||
turkey.updateLayerTemps(); |
||||
} |
||||
|
@ -0,0 +1,233 @@
@@ -0,0 +1,233 @@
|
||||
/* Screens, inheritance would be nice */ |
||||
function LoadingTitleScreen( stage, gameState ){ |
||||
var that = this; |
||||
this.picture = new createjs.Bitmap( "res/Loading-Title.png" ); |
||||
this.ovenLight = new createjs.Shape(); |
||||
this.ovenLight.graphics.beginFill( "red" ).drawCircle( 396, 318, 5 ); |
||||
this.ovenLight.addEventListener( "click", function(){alert("hello world")}); |
||||
|
||||
stage.addChild( this.picture ); |
||||
stage.addChild( this.ovenLight ); |
||||
|
||||
this.uiElems = []; |
||||
this.uiElems.push( new DialogUI( stage ) ); |
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
||||
function InfoHelpScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Main.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
this.uiElems = []; |
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
|
||||
|
||||
} |
||||
|
||||
function MainScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Main.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
// buttons info/credits/start
|
||||
var infoButton = new createjs.Shape(); |
||||
infoButton.graphics.beginFill("#ffffff").drawRect(13, 445, 222, 65); |
||||
infoButton.alpha = 0.1; |
||||
infoButton.addEventListener( "click", function(){ gameState.pubsub.publish( "SwitchScreen", "InfoHelpScreen" ) } ); |
||||
|
||||
var creditsButton = new createjs.Shape(); |
||||
creditsButton.graphics.beginFill("#ffffff").drawRect(13, 515, 222, 65); |
||||
creditsButton.alpha = 0.1; |
||||
creditsButton.addEventListener( "click", function(){ gameState.pubsub.publish( "SwitchScreen", "CreditsScreen" ) } ); |
||||
|
||||
var startButton = new createjs.Shape(); |
||||
startButton.graphics.beginFill("#ffffff").drawRect(564, 520, 222, 65); |
||||
startButton.alpha = 0.1; |
||||
startButton.addEventListener( "click", function(){ gameState.pubsub.publish( "SwitchScreen", "DifficultyScreen" ) } ); |
||||
|
||||
stage.addChild( infoButton ); |
||||
stage.addChild( creditsButton ); |
||||
stage.addChild( startButton ); |
||||
|
||||
this.uiElems = []; |
||||
|
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
|
||||
//start button
|
||||
|
||||
} |
||||
|
||||
function DifficultyScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Difficulty-Selection.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
var easyButton = new createjs.Shape(); |
||||
easyButton.graphics.beginFill("#ffffff").drawRect(170, 40, 450, 105); |
||||
easyButton.alpha = 0.1; |
||||
easyButton.addEventListener( "click", function(){ gameState.pubsub.publish( "SwitchScreen", "KitchenScreen" ) } ); |
||||
|
||||
var hardButton = new createjs.Shape(); |
||||
hardButton.graphics.beginFill("#ffffff").drawRect(170, 150, 450, 105); |
||||
hardButton.alpha = 0.1; |
||||
hardButton.addEventListener( "click", function(){ gameState.pubsub.publish( "SwitchScreen", "KitchenScreen" ) } ); |
||||
|
||||
stage.addChild( easyButton ); |
||||
stage.addChild( hardButton ); |
||||
|
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
||||
function KitchenScreen( stage, gameState ){ |
||||
var that = this; |
||||
this.uiElems = []; |
||||
|
||||
this.uiElems.push( new OvenUI( stage ) ); |
||||
this.uiElems.push( new ClockUI( stage, gameState ) ) |
||||
this.uiElems.push( new DialogUI( stage ) ); |
||||
|
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
||||
function MarketScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Main.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
this.uiElems = []; |
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
|
||||
|
||||
} |
||||
|
||||
function TurkeyOutScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Main.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
this.uiElems = []; |
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
|
||||
|
||||
} |
||||
|
||||
function EndingScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Main.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
this.uiElems = []; |
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
|
||||
|
||||
} |
||||
|
||||
function ScoreScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Main.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
this.uiElems = []; |
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
|
||||
// Retry Button
|
||||
} |
||||
|
||||
function CreditsScreen( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.background = new createjs.Bitmap( "res/Main.png" ); |
||||
stage.addChild( this.background ); |
||||
|
||||
this.uiElems = []; |
||||
return { |
||||
blit : function(){ |
||||
|
||||
// Draw all the uiElements
|
||||
for( var index in that.uiElems ){ |
||||
that.uiElems[ index ].tick(); |
||||
} |
||||
} |
||||
} |
||||
//
|
||||
} |
@ -0,0 +1,79 @@
@@ -0,0 +1,79 @@
|
||||
function ClockUI( stage, gameState ){ |
||||
var that = this; |
||||
|
||||
this.minuteRadius = 40; |
||||
this.hourRadius = 0.7 * this.minuteRadius; |
||||
this.clockX = 300; |
||||
this.clockY = 100; |
||||
|
||||
this.getClockAngles = function( ){ |
||||
var currTime = new Date( gameState.currentTime ); |
||||
var hourAngle = 720 * ( currTime.getHours() / 24 ) - 90; |
||||
var minuteAngle = 360 * ( currTime.getMinutes() / 60 ) - 90; |
||||
return [ hourAngle, minuteAngle ]; |
||||
} |
||||
|
||||
var minuteLine = new createjs.Shape(); |
||||
minuteWidth = this.minuteRadius; |
||||
minuteHeight = 1; |
||||
minuteLine.graphics.beginFill('black').drawRect( 0, 0, minuteWidth, minuteHeight ); |
||||
minuteLine.regX = 0; |
||||
minuteLine.regY = minuteHeight / 2; |
||||
minuteLine.x = this.clockX; |
||||
minuteLine.y = this.clockY; |
||||
|
||||
var hourLine = new createjs.Shape(); |
||||
hourWidth = this.hourRadius; |
||||
hourHeight = 1; |
||||
hourLine.graphics.beginFill('black').drawRect( 0, 0, hourWidth, hourHeight ); |
||||
hourLine.regX = 0; |
||||
hourLine.regY = hourHeight / 2; |
||||
hourLine.x = this.clockX; |
||||
hourLine.y = this.clockY; |
||||
|
||||
stage.addChild( minuteLine ); |
||||
stage.addChild( hourLine ); |
||||
return { |
||||
tick: function(){ |
||||
var angles = that.getClockAngles(); |
||||
hourLine.rotation = angles[0]; |
||||
minuteLine.rotation = angles[1]; |
||||
} |
||||
} |
||||
|
||||
} |
||||
|
||||
function OvenUI( stage ){ |
||||
var that = this; |
||||
|
||||
this.ovenLight = new createjs.Shape(); |
||||
this.analogClock = ""; |
||||
this.text = new createjs.Text( "325F", "50px Arial", "#ff7700" ); |
||||
this.text.x = 70; |
||||
this.text.y = 100; |
||||
this.text.textBaseline = "alphabetic"; |
||||
|
||||
//Create a Shape DisplayObject.
|
||||
this.circle = new createjs.Shape(); |
||||
this.circle.graphics.beginFill( "red" ).drawCircle( 0, 0, 40 ); |
||||
this.ovenLight.graphics.beginFill( "red" ).drawCircle( 223, 73, 5 ); |
||||
|
||||
//Set position of Shape instance.
|
||||
this.circle.x = this.circle.y = 50; |
||||
|
||||
this.picture = new createjs.Bitmap( "res/Base_Game_Screen.png" ); |
||||
//this.picture.scaleX = this.picture.scaleY = 0.5;
|
||||
stage.addChild( this.picture ); |
||||
stage.addChild( this.circle ); |
||||
stage.addChild( this.ovenLight ); |
||||
stage.addChild( this.text ); |
||||
return { |
||||
tick: function(){ |
||||
// Circle will move 10 units to the right.
|
||||
that.circle.x += 1; |
||||
|
||||
// Will cause the circle to wrap back
|
||||
if ( that.circle.x > stage.canvas.width ) { that.circle.x = 0; } |
||||
} |
||||
} |
||||
} |
Loading…
Reference in new issue